In Solidity, you can inherit from multiple contracts, but the order of inheritance is crucial. An incorrect inheritance order can lead to issues such as function ambiguity and storage layout conflicts. This ambiguity is related to the Diamond Problem, where two base contracts have functions with the same name, leading to confusion about which one should be prioritized. Solidity has a structured way of handling these issues, but it requires developers to understand and correctly apply the inheritance rules.
Consider a scenario where you have three contracts:
BaseContractA, BaseContractB, and DerivedContract. Both BaseContractA and BaseContractB define a function named foo(). If DerivedContract inherits from both BaseContractA and BaseContractB, the order in which these base contracts are listed in the inheritance list determines which foo() function gets called.
For example, if DerivedContract inherits from BaseContractA first and then BaseContractB like this:
Consider a scenario where you have three contracts:
BaseContractA, BaseContractB, and DerivedContract. Both BaseContractA and BaseContractB define a function named foo(). If DerivedContract inherits from both BaseContractA and BaseContractB, the order in which these base contracts are listed in the inheritance list determines which foo() function gets called.
For example, if DerivedContract inherits from BaseContractA first and then BaseContractB like this:
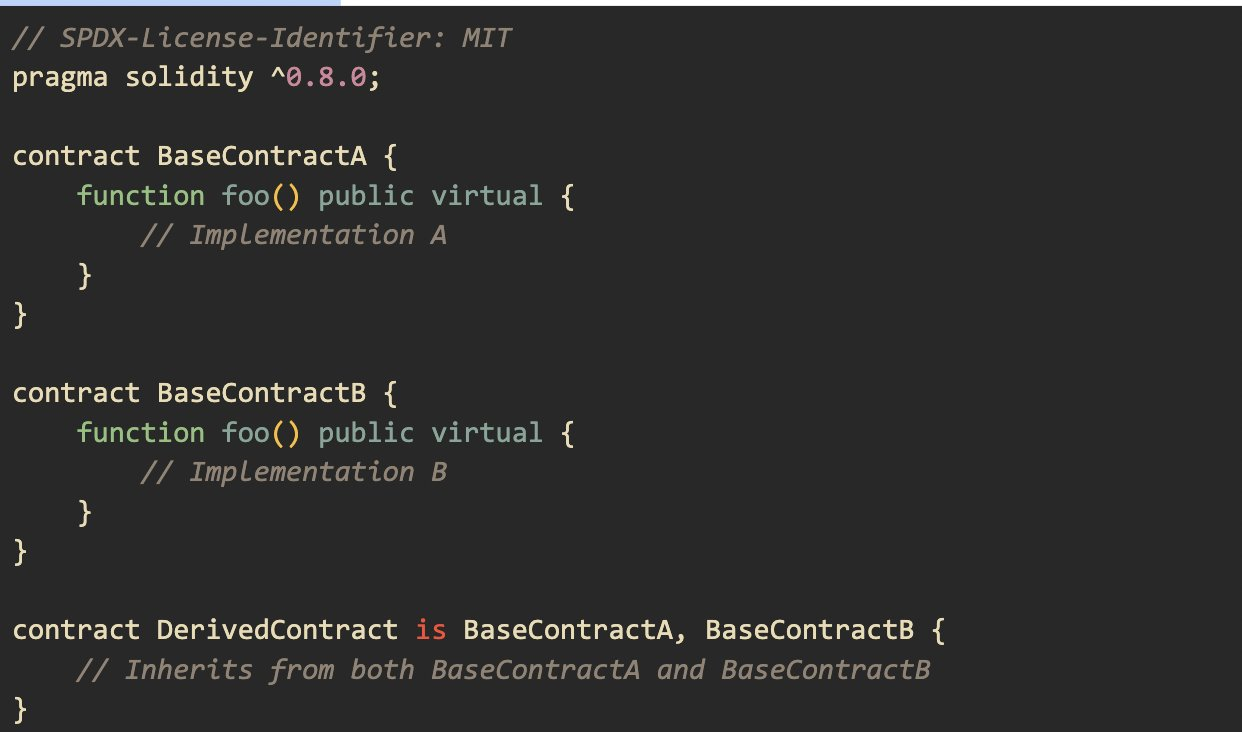
When you call foo() on an instance of DerivedContract, the foo() function from BaseContractB will be executed because BaseContractB is listed last in the inheritance list.
Conversely, if the inheritance order is reversed:

Now, calling foo() on an instance of DerivedContract will execute the foo() function from BaseContractA, as it is listed last in the inheritance list.
This behavior is due to Solidity's method resolution order (MRO), which follows a linearization process called C3 linearization. This ensures a deterministic and consistent method resolution order in the presence of multiple inheritance, but it requires developers to be aware of the inheritance order to avoid unexpected behavior.