Override Logic and Its Significance in Smart Contract Auditing
Introduction
In Solidity, override logic is a fundamental concept that comes into play when a contract inherits functions from one or more base contracts and provides its own implementation. For auditors, understanding how overrides work—and how Solidity determines which implementation to call—is critical for detecting logical flaws, security vulnerabilities, and potential misconfigurations in complex inheritance hierarchies.
This post will explore the mechanics of override logic, using code snippets and images that illustrate inheritance structures in action. We’ll also highlight how to securely implement and audit override logic, ensuring that your contracts behave as intended. For professional assistance in auditing and reviewing your Solidity code, consider Bailsec’s audit services.
1. Basic Override Mechanism
When a derived contract overrides a function defined in a base contract, it must maintain the same function signature and use the override keyword:
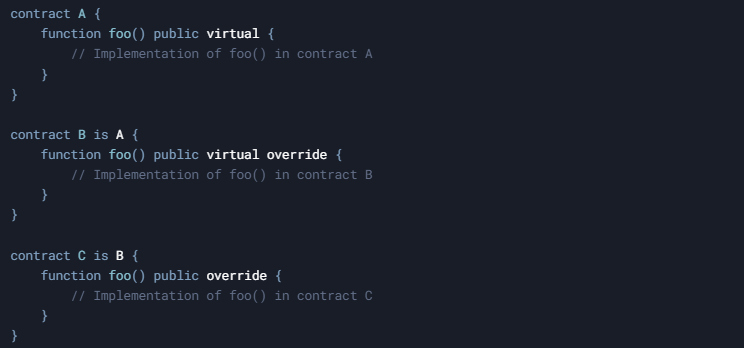
How Solidity Resolves Calls
When calling foo() on an instance of C, Solidity starts at C and moves up the inheritance chain until it finds an implementation. Here’s the resolution order:
- Contract C: If C has an override of foo(), use it.
- Contract B: If C doesn’t override foo(), check B.
- Contract A: If neither C nor B override foo(), fallback to A.
This methodical search ensures the most derived implementation is always used first. If a function isn’t overridden in a derived contract, Solidity looks to the parent.
2. Extending Base Implementations with super
Solidity’s override system also allows you to extend rather than fully replace a base function by calling the parent contract’s implementation via super. This is particularly helpful when you need to preserve some of the original logic:
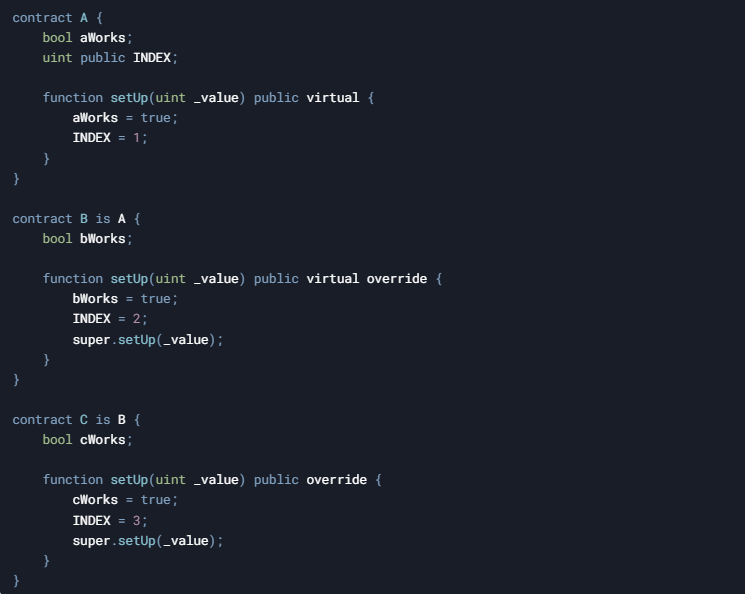
In this example:
- Contract C calls its own logic, sets INDEX to 3, and then calls super.setUp(_value), which references B’s implementation.
- Contract B sets its own state and INDEX to 2, then calls super.setUp(_value) referencing A’s implementation.
- Contract A sets its own state and INDEX to 1.
This chain of calls ensures all relevant logic is executed in the correct order. During audits, you must verify that these super calls do not introduce unexpected behavior or reentrancy risks.
3. Security Implications and Audit Focus
a. Inheritance Misconfiguration
When multiple contracts share function names and inheritance is complex, it’s easy to misconfigure overrides. A derived contract might unintentionally omit the override keyword, leading to unexpected calls or partial implementations.
Audit Tip: Confirm that each function meant to be overridden is marked as virtual in the base and override in the child. Ensure no accidental shadowing or name conflicts occur.
b. Incomplete or Missing super Calls
If a derived contract fails to call super when needed, it may skip crucial logic in the base contract. This can lead to partial initialization, missed event emissions, or incomplete state updates.
Audit Tip: Check whether the derived contract’s override logic is meant to extend or replace the base function. If extension is intended, verify the presence and correctness of super calls.
c. Upgradeable Contracts
In upgradeable contract systems (e.g., using proxy patterns), override logic can become even more critical. The inherited functions must remain compatible across upgrades, and any changes in function signatures can break the proxy’s storage layout or function resolution.
Audit Tip: Confirm that any modifications to override logic maintain backward compatibility. For more guidance on upgradeable patterns, refer to Bailsec’s workflow documentation.
4. Best Practices for Override Logic
- Mark All Overridable Functions:
- Use the virtual keyword for any function that might be overridden in derived contracts.
- Use override Correctly:
- Ensure derived contracts explicitly declare which base functions they’re overriding to avoid ambiguity.
- Call super When Needed:
- If you need the parent’s logic, call super at the appropriate point in your function.
- Keep Inheritance Hierarchies Simple:
- Deep or multiple inheritance can lead to confusion and potential vulnerabilities.
- Comprehensive Testing:
- Write tests that exercise each level of inheritance to confirm the correct function implementations are invoked.
For in-depth reviews and guidance on override logic, explore Bailsec’s services.
Conclusion
Override logic in Solidity is a powerful feature that enables flexible, modular contract design. However, with flexibility comes complexity—inheritance chains can be hard to track, and missing or incorrect overrides can lead to security vulnerabilities or unexpected behavior. As an auditor, it’s essential to verify that each override is intentional, properly marked, and tested.
By understanding how Solidity resolves overridden functions, how to use super to extend base logic, and how these features can impact upgradeable contracts, you’ll be better equipped to identify risks and ensure robust, maintainable code. For further resources on smart contract security, consider reading more on Bailsec’s blog or consulting the experts at Bailsec for a professional audit.
Disclaimer: This article is intended for informational purposes only and does not constitute legal or financial advice. Always conduct thorough testing and consider professional audits to ensure the security of your smart contracts.