Smart contracts can receive Ether through different mechanisms, either via direct transfers or by being called with data that doesn't match any existing function signature. Solidity has two specific functions to handle these scenarios:
receive() Function:
This function is triggered when a contract receives Ether without any accompanying data. It’s used solely to receive and manage Ether payments.
If a contract doesn’t have a receive() function but has a fallback() function, the fallback() will handle the Ether transfer.
receive() Function:
This function is triggered when a contract receives Ether without any accompanying data. It’s used solely to receive and manage Ether payments.
If a contract doesn’t have a receive() function but has a fallback() function, the fallback() will handle the Ether transfer.
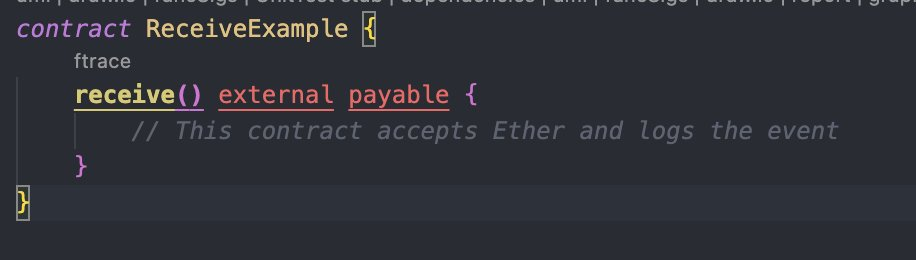
fallback() Function:
This function is triggered when the contract is called with data that does not match any existing function signature, or when Ether is sent without a matching function but no receive() function exists.
It can be designed to handle these unrecognized calls, or in some cases, reject them.
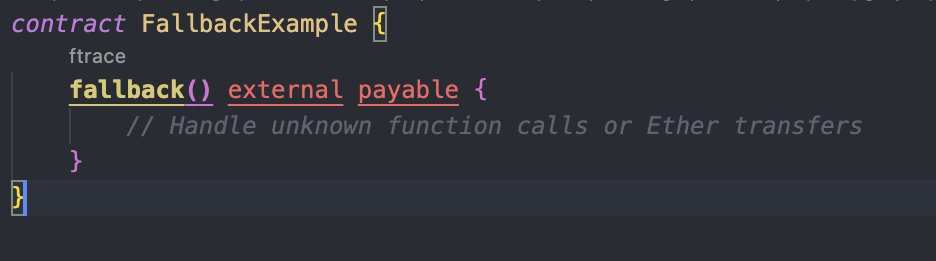
Denying Receipt of Ether with a Manipulated fallback()
In some scenarios, contracts might want to reject incoming Ether transfers. For example, a contract might not want to receive Ether under certain conditions, such as after a certain balance is reached or if the transfer is unauthorized. This can be done by using the revert() statement in the fallback() or receive() functions, which effectively prevents the contract from accepting Ether.
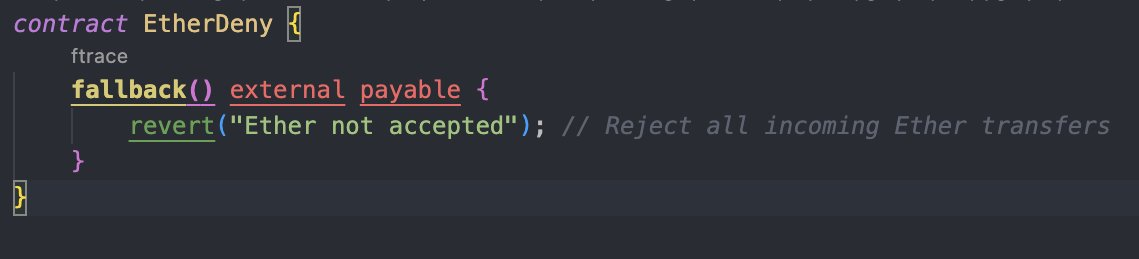
Scenario: DoS via Failed Refunds
Imagine a contract that performs an action and needs to refund Ether to users after the operation is complete. The contract expects the refund to succeed, but if the receiving contract’s fallback function is manipulated to reject the refund, the refund operation fails. This can trigger a Denial of Service (DoS) attack, where the contract becomes stuck and unable to process further transactions because it cannot successfully refund the Ether.