In Solidity, modifiers and Private functions are commonly used to restrict access to certain functionalities within a smart contract. However, they differ in their implementation and usage.
Modifiers
Modifiers in Solidity are special functions that can be appended to other functions to add additional functionality or constraints to them. They are commonly used to enforce access control by requiring certain conditions to be met before allowing the execution of a function.
Modifiers
Modifiers in Solidity are special functions that can be appended to other functions to add additional functionality or constraints to them. They are commonly used to enforce access control by requiring certain conditions to be met before allowing the execution of a function.

Modifiers offer a convenient way to enforce access control without duplicating code across multiple functions.
They promote code reusability and maintainability by allowing developers to define access control logic in one place and apply it to multiple functions throughout the contract. When a modifier is applied to a function, the compiler essentially expands the code of the modifier into the function itself during compilation.
This means that each function that uses the modifier will include a copy of the modifier code. However, it's important to note that this does not significantly impact the size of the deployed contract on the Ethereum blockchain.
The code is ultimately stored in bytecode form, and the size difference between using modifiers and private functions is negligible in practice.
Private Functions
On the other hand, private functions in Solidity are regular functions that are restricted to be called only from within the contract that defines them. They cannot be accessed or invoked externally or by other contracts. Private functions are useful for encapsulating logic that should only be accessible within the contract and are not intended for external interaction. Here's how a private function is defined in Solidity:

Since private functions are not directly callable from outside the contract, they are not included in the contract's external interface.
However, they do still occupy space within the contract's bytecode. Private functions are included in the contract's bytecode to facilitate internal function calls.
Thus, the argument that private functions save space compared to modifiers is not entirely accurate. Both modifiers and private functions contribute to the size of the contract bytecode, but the difference in size is typically negligible.
When to Use Modifiers Vs. When to Use Private Functions
Knowing when to use modifiers versus private functions in Solidity depends on the specific requirements and design considerations of your smart contract. Here are guidelines for when to use each, along with corresponding code snippets:
Use Modifiers When:
Reusability: You need to enforce the same access control logic across multiple functions within your contract.
Readability: You want to clearly indicate access control requirements directly within the function signature.
Reducing Redundancy: You want to avoid duplicating access control logic across multiple functions.
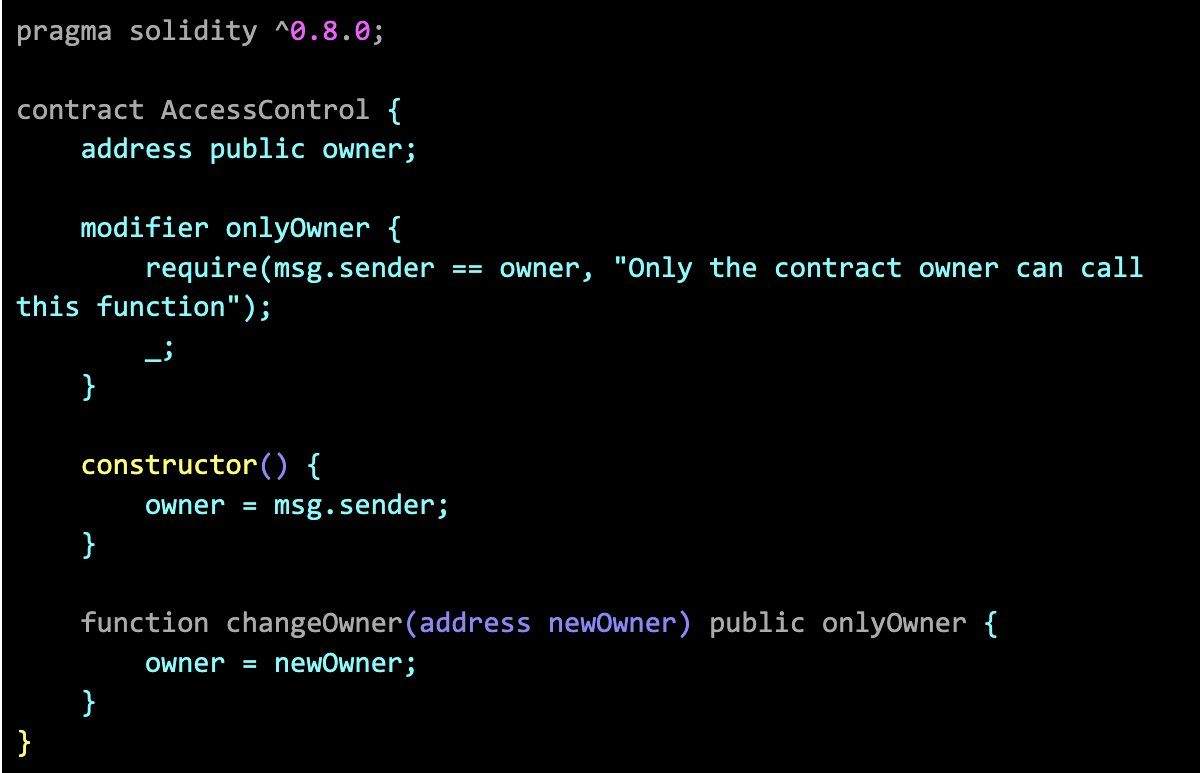
In this example, the onlyOwner modifier restricts access to functions to only the contract owner. It is applied to the changeOwner function, ensuring that only the owner can change the contract ownership.
Use Private Functions When:
Encapsulation: You want to encapsulate internal logic that is not intended to be accessed externally or by other contracts.
Internal Operations: You need to perform specific operations that are not meant to be exposed to external parties.
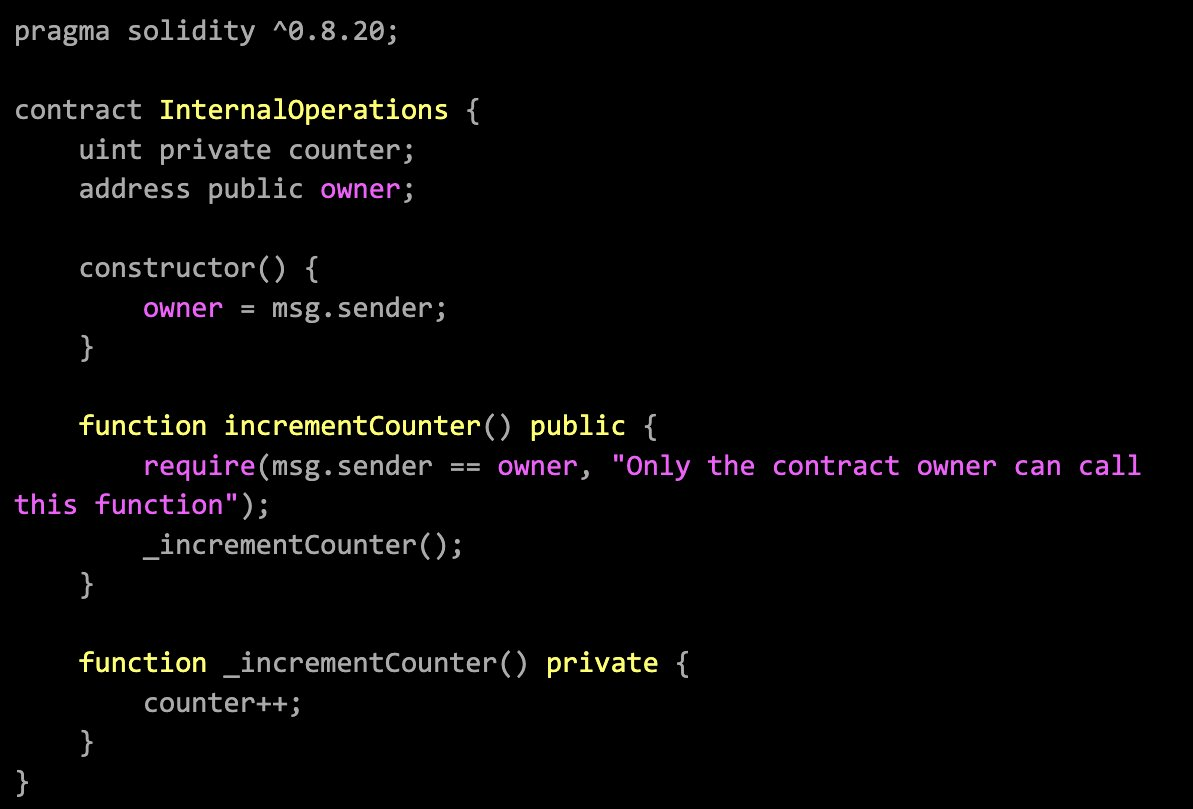
In this example, the _incrementCounter function is marked as private, indicating that it is only meant to be accessed internally within the contract. It encapsulates the logic to increment the counter and is called by the incrementCounter function, which enforces access control using a require statement.
Link to the article
https://twitter.com/CharlesWangP/status/1777661696775016551