Modifiers serve as specialized functions designed to alter the behavior of other functions within a Solidity smart contract. They are particularly useful for enforcing conditions or executing additional logic before allowing a function to execute. Modifiers significantly streamline Solidity code by reducing the need for repetitive code segments and enhancing code readability.
It's essential to note that while modifiers are powerful tools for enhancing contract logic, they do have limitations. Specifically, modifiers cannot directly modify a contract's storage, including structs.
Here's how to define and apply a modifier in Solidity:
It's essential to note that while modifiers are powerful tools for enhancing contract logic, they do have limitations. Specifically, modifiers cannot directly modify a contract's storage, including structs.
Here's how to define and apply a modifier in Solidity:

Inside the modifier, there's a `require` statement. This statement checks if the address that called the function (msg.sender) is equal to the owner of the contract. If it's not, the transaction will be reverted, and any state changes made so far will be undone.

With the onlyOwner modifier applied here above, if anyone else tries to call this function, the `require` statement inside the modifier will fail, and the transaction will be reverted, preventing unauthorized users from changing the owner of the contract.
What Does the _; Symbol mean?
When you define a modifier, it's like creating a set of rules or conditions that a function must meet before it can execute. These rules are placed within the body of the modifier. After defining the rules, Solidity needs to know where to insert the actual function code. This is where the _; comes into play.

1. Gate Checks
Consider a voting system contract where a gate check ensures only eligible voters can cast their ballots. The modifier verifies if the caller's address is listed in the registered voter database before permitting them to submit their vote. If the caller is not registered, the gate check prevents the function from executing, preserving the integrity of the voting process.
2. Prerequisites
Unlike gate checks, prerequisites focus on setting up the requisite environment for function execution rather than checking if a certain condition is true. Suppose you're building a decentralized marketplace where users can list items for sale. A prerequisite modifier ensures that sellers have sufficient inventory of the items they wish to list before allowing them to publish the listing.
3. Reentrancy Attack Prevention
Reentrancy attacks exploit recursive function calls to maliciously manipulate smart contracts. To mitigate such attacks, a modifier can be employed to scrutinize function calls to ensure they aren't recursive.
How to Inherit and Override Modifiers
Modifier overriding in Solidity allows for the customization of inherited modifiers in derived contracts. By marking a modifier as "virtual" in the base contract, derived contracts gain the ability to override the behavior of that modifier.
However, in order for the derived contract to automatically gain access to all the functions, variables, and modifiers defined in the base contract, we need to use inheritance. It allows us to add new modifiers or override existing ones. To inherit a modifier, use the is keyword in the contract that you want to inherit the modifier from.
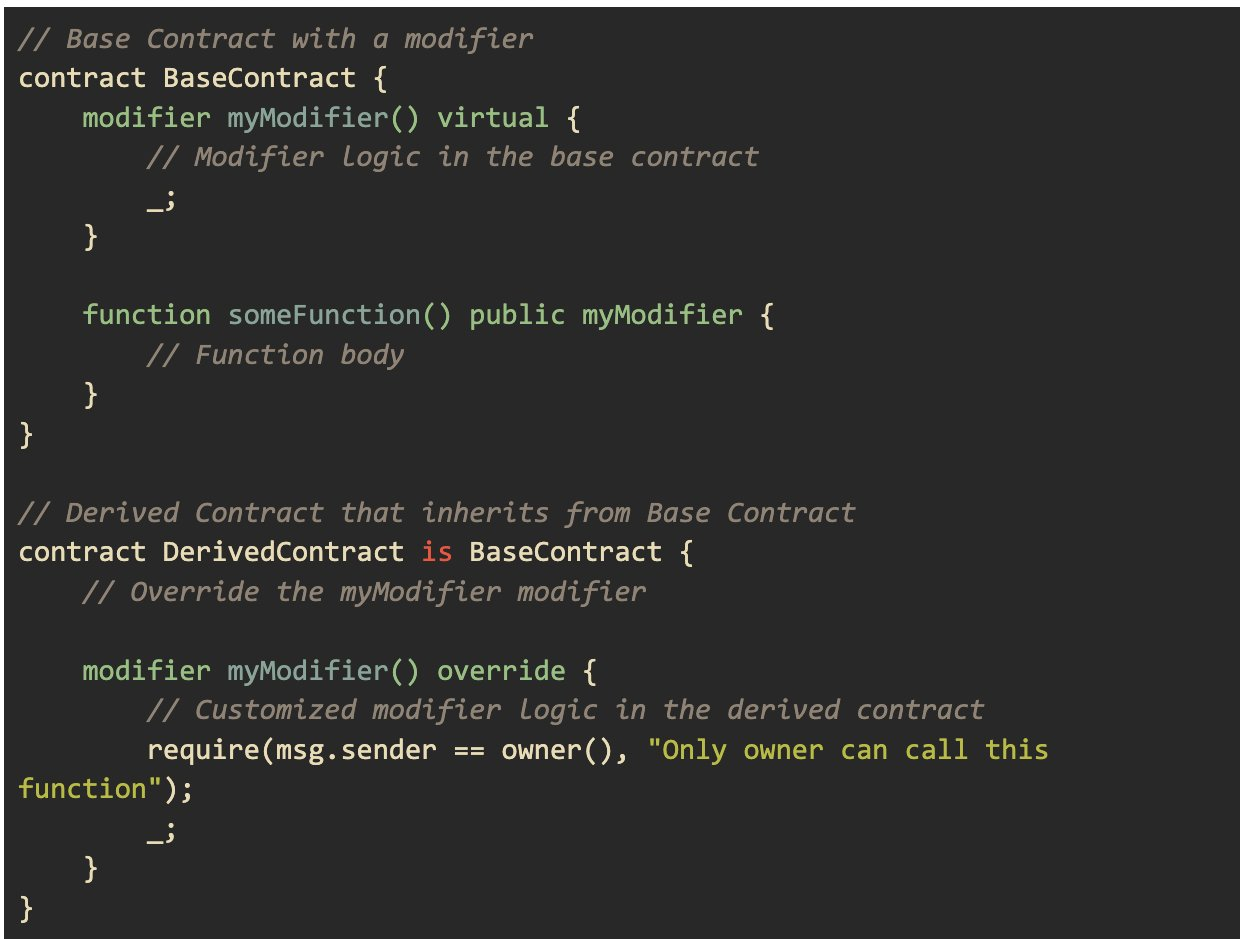
Multiple Modifiers and their Order
Solidity permits the application of multiple modifiers to a single function. This enables developers to impose multiple conditions or execute diverse sets of logic before allowing a function to execute. To apply multiple modifiers to a function, simply separate them with commas in the function declaration.
function myFunction() public modifier1, modifier2 {...}
Importantly, the order in which modifiers are listed matters. When multiple modifiers are applied to a function, they are executed sequentially in the order they are listed.

Link to the article
https://twitter.com/CharlesWangP/status/1782748471432188326