When a contract inherits from another, it inherits all of its state variables and functions. However, if the child contract declares a state variable with the same name, it doesn't override the inherited variable; instead, it attempts to create a new, separate variable that shadows the inherited one. In Solidity, state variables cannot be directly overridden by re-declaring them. Instead, shadowing occurs when the child contract introduces a new state variable with the same name, effectively creating a new variable that shadows the inherited one.
In summary, attempting to shadow state variables by re-declaring them in a derived contract will result in a compilation error.
Here is an example of incorrect code that will result in a compilation error due to shadowing:
In summary, attempting to shadow state variables by re-declaring them in a derived contract will result in a compilation error.
Here is an example of incorrect code that will result in a compilation error due to shadowing:
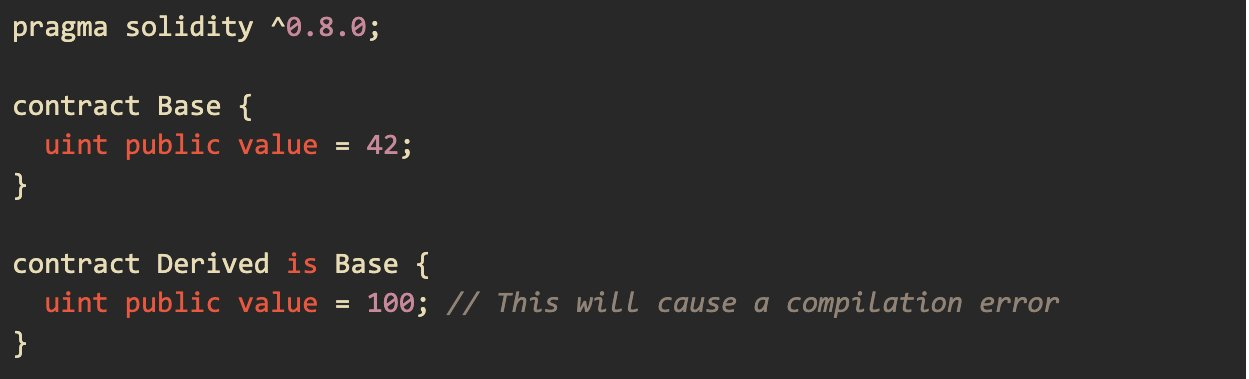
This means that the base contract's variable will become inaccessible through the derived contract's context if shadowing were allowed..
Correct Approach to Modify Inherited State Variables
To correctly modify an inherited state variable, you should not declare a new variable with the same name. Instead, you should assign a new value to the inherited variable within the child contract's constructor or another function. This approach ensures that the inherited state variable's value is modified as intended, allowing for clear and predictable contract behavior.
Here’s the correct approach:
Modifying the inherited variable in the constructor:
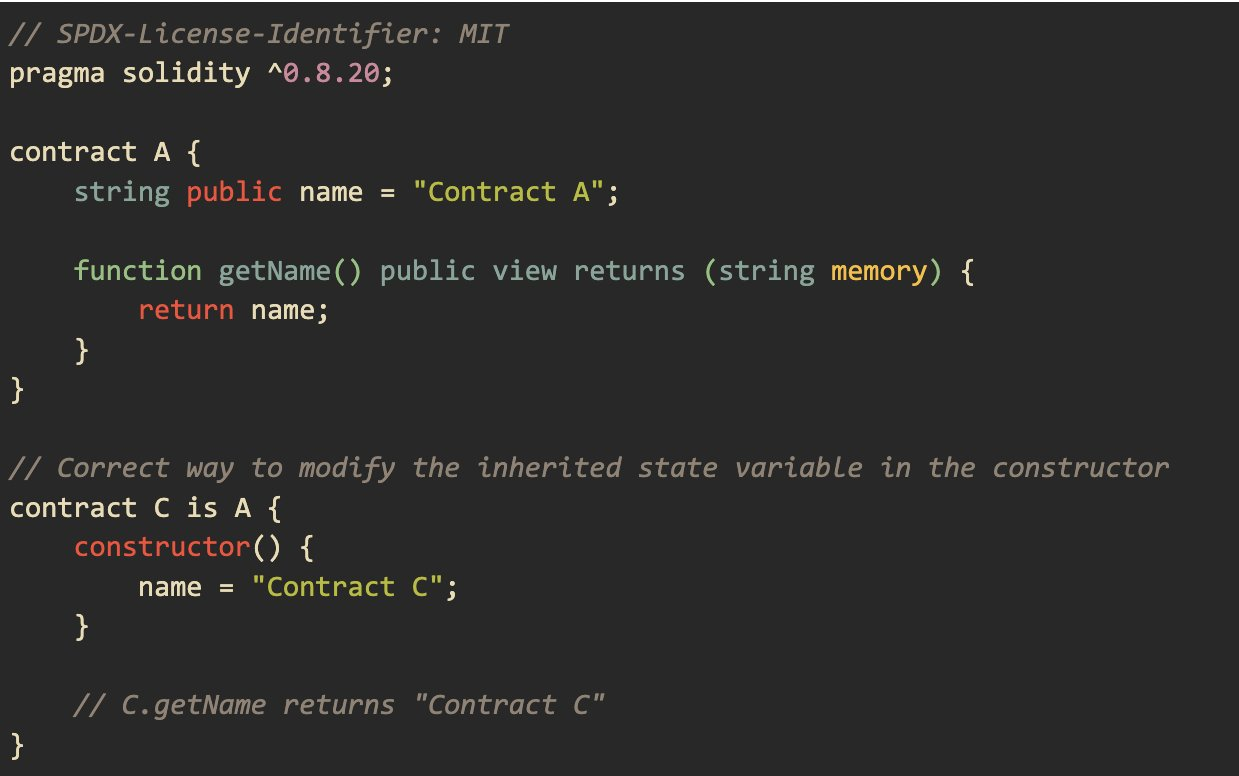
Inheritance and Shadowing: State variables in Solidity cannot be overridden by re-declaring them in a derived contract. Attempting to do so results in a compilation error.
Correct Approach: To modify an inherited state variable, assign a new value to it within the derived contract’s constructor or another function. This ensures the inherited state variable's value is modified as intended without causing any compilation errors.