The “call” function in Solidity is a low-level function that enables contract-to-contract communication by invoking functions or sending Ether to a specified contract address. It operates by taking calldata and executing it on the intended recipient via the low-level EVM opcode CALL.
`call` is used to call the `fallback` and `receive` functions of the contract. Receive is called when no data is sent in the function call, and ether is sent. The fallback function is called when no function signature matches the call.
`call` is used to call the `fallback` and `receive` functions of the contract. Receive is called when no data is sent in the function call, and ether is sent. The fallback function is called when no function signature matches the call.
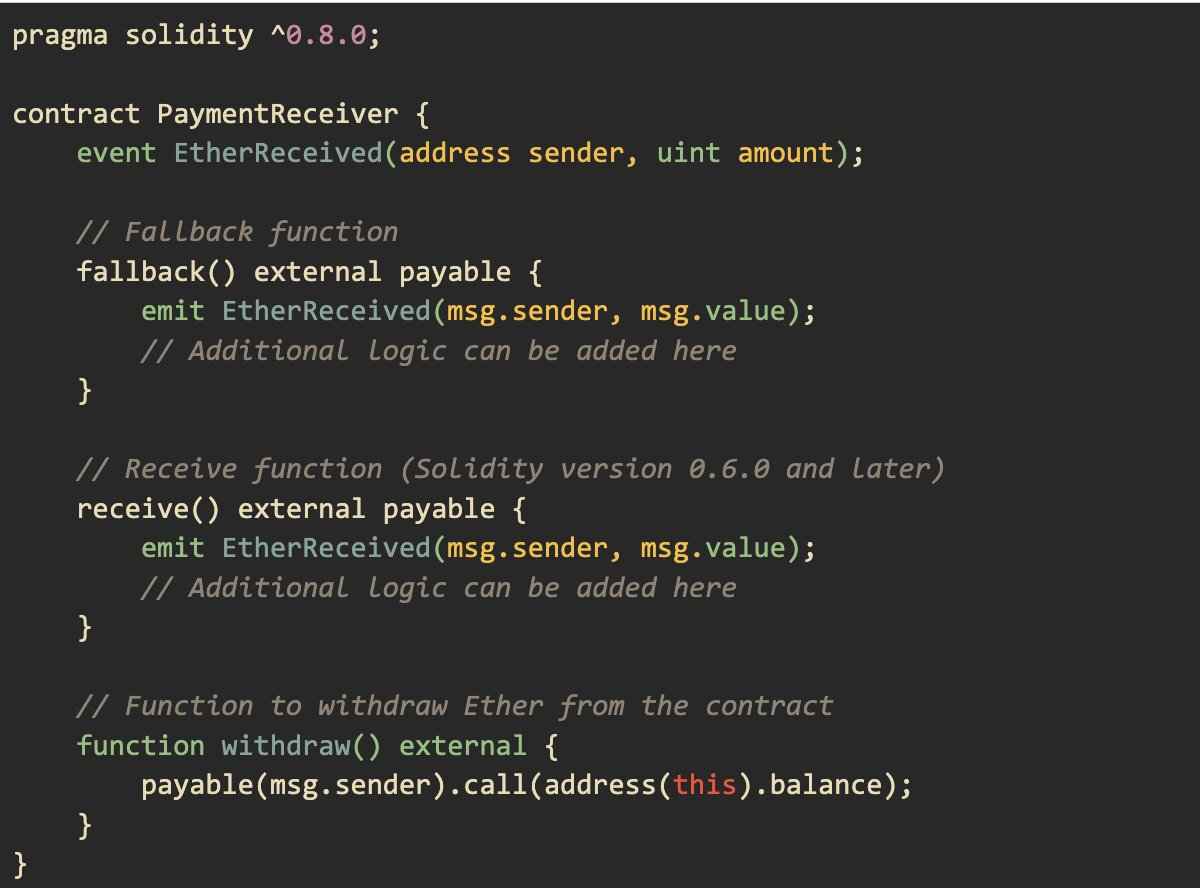
When using the call method, the called function is seen as a part of another smart contract. However, the called function will use the caller's storage, msg.sender, and msg.value.
So when making a call, users can specify the target contract address and target function with other parameters. The amount of Ether to send and the execution gas limit can also be defined.
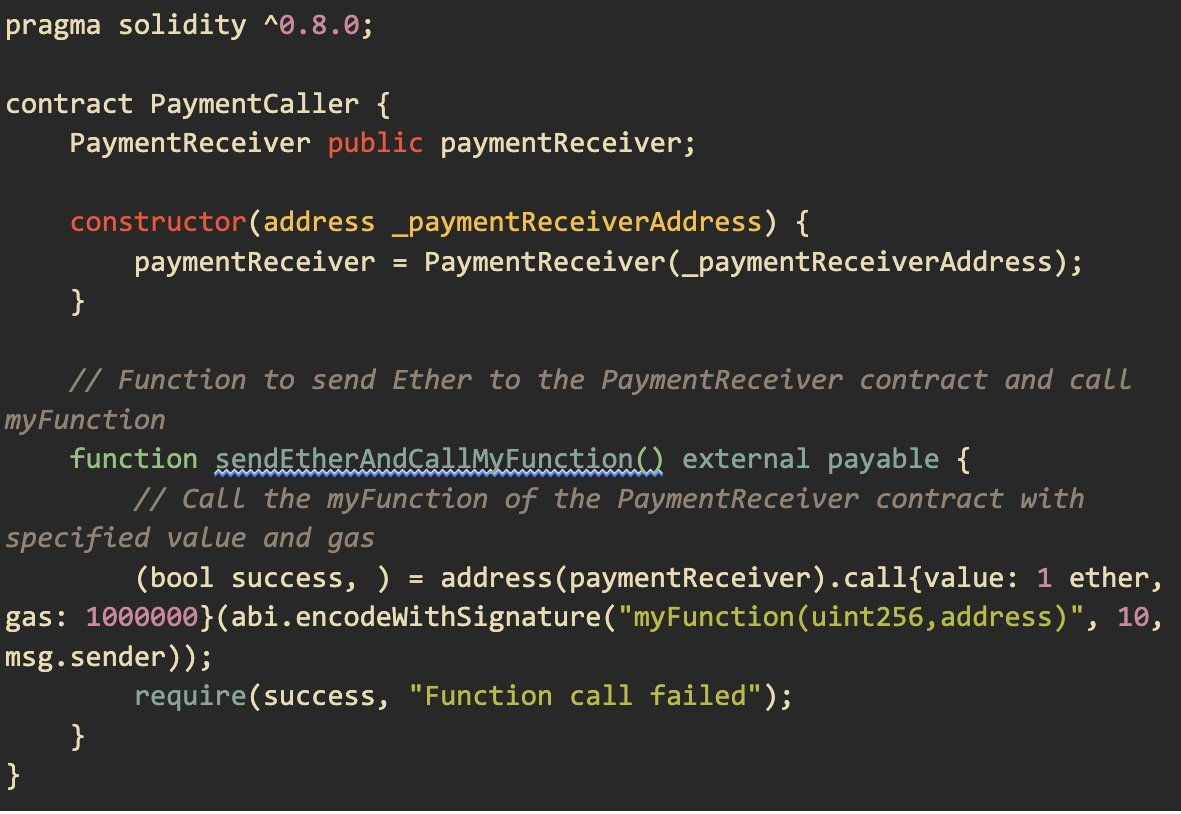
In the snippet above, the sendEtherAndCallMyFunction function allows users to send Ether to the PaymentReceiver contract and call its myFunction method. Within this function, we use the call function to execute the myFunction method of the PaymentReceiver contract. We specify the value to send (1 ether) and the amount of gas to provide (1000000).
Recommendations for Call Functions.
It is generally discouraged to use the call function for invoking contract functions in most scenarios. This is because call bypasses important checks such as type validation and function existence verification, which can lead to unexpected behavior or vulnerabilities in the contract.
When a function is called using `call`, any revert that occurs within the called function does not propagate back to the calling contract. As a result, the calling contract is not aware of the revert and may continue executing erroneously. Additionally, if `call` is used to invoke a function that does not exist or has been renamed, it will trigger the fallback function instead. This can lead to unintended behavior if the fallback function is not designed to handle such calls appropriately.
Call vs. Delegatecall
The main difference between `delegatecall` and `call` functions in Solidity lies in how they execute the called function and handle the state. Here's a breakdown:
Syntax and Options:
Both `delegatecall` and call have similar syntax.
The `call` function accepts both value (amount of Ether) and gas options.
The `delegatecall` function does not accept the value option; it only accepts gas.
Execution Context:
delegatecall executes the called function within the context of the calling contract. This means the called function shares the same storage context and state variables as the calling contract. It's as if the code of the called function is part of the calling contract.
on the other hand, call function executes the called function in its original context as if it were part of another smart contract. This means that the called function operates on its own storage and state variables, separate from the calling contract.
Use Cases:
`delegatecall` is often used for proxy contracts or library contracts, where the calling contract wants to delegate certain functionality to another contract but still maintains control over its own state.
`call` is typically used for standard contract-to-contract interactions where the calling contract needs to interact with another contract's functions without affecting its own state.