Before-After Pattern explained:
Transfer-tax tokens are tokens that apply a fee whenever a transfer is made. This means that the recipient does not receive the full amount specified in the transfer, as a portion is deducted as a tax or fee. For example, if 100 tokens are transferred with a 5% tax, the recipient only receives 95 tokens, while the remaining 5 tokens are taken as a fee, often redistributed to holders, burned, or directed to another purpose.
The Issue with Staking Contracts and Transfer-Tax Tokens
In staking contracts, users transfer tokens to the contract, and the contract typically updates the user's staking balance by the amount transferred. However, in the case of transfer-tax tokens, the contract records the full transfer amount but receives less due to the applied tax. As a result, the contract will incorrectly account for more tokens than it actually holds, leading to potential accounting errors and unexpected behavior when users try to withdraw their stake.
Example: If a user stakes 100 tokens and there is a 5% transfer tax, the contract may assume it received the full 100 tokens, but it actually only received 95. Over time, this discrepancy can cause problems, such as overestimating the tokens available for withdrawal.
The Fix: Cache Balance and Calculate the Actual Amount Received
To solve this issue, you can modify the staking contract to cache the contract's balance before and after the transfer. By calculating the difference between the pre-transfer and post-transfer balance, the contract can correctly determine how many tokens were actually received, regardless of any transfer tax.
Additionally, since this method breaks the Check-Effects-Interaction (CEI) pattern, adding a reentrancy guard is necessary to protect against potential reentrancy attacks.
Cache the contract's balance before the transfer
Perform the token transfer
Calculate the actual amount received by subtracting the cached balance from the new balance
Update the staking balance using the actual amount received
Transfer-tax tokens are tokens that apply a fee whenever a transfer is made. This means that the recipient does not receive the full amount specified in the transfer, as a portion is deducted as a tax or fee. For example, if 100 tokens are transferred with a 5% tax, the recipient only receives 95 tokens, while the remaining 5 tokens are taken as a fee, often redistributed to holders, burned, or directed to another purpose.
The Issue with Staking Contracts and Transfer-Tax Tokens
In staking contracts, users transfer tokens to the contract, and the contract typically updates the user's staking balance by the amount transferred. However, in the case of transfer-tax tokens, the contract records the full transfer amount but receives less due to the applied tax. As a result, the contract will incorrectly account for more tokens than it actually holds, leading to potential accounting errors and unexpected behavior when users try to withdraw their stake.
Example: If a user stakes 100 tokens and there is a 5% transfer tax, the contract may assume it received the full 100 tokens, but it actually only received 95. Over time, this discrepancy can cause problems, such as overestimating the tokens available for withdrawal.
The Fix: Cache Balance and Calculate the Actual Amount Received
To solve this issue, you can modify the staking contract to cache the contract's balance before and after the transfer. By calculating the difference between the pre-transfer and post-transfer balance, the contract can correctly determine how many tokens were actually received, regardless of any transfer tax.
Additionally, since this method breaks the Check-Effects-Interaction (CEI) pattern, adding a reentrancy guard is necessary to protect against potential reentrancy attacks.
Cache the contract's balance before the transfer
Perform the token transfer
Calculate the actual amount received by subtracting the cached balance from the new balance
Update the staking balance using the actual amount received
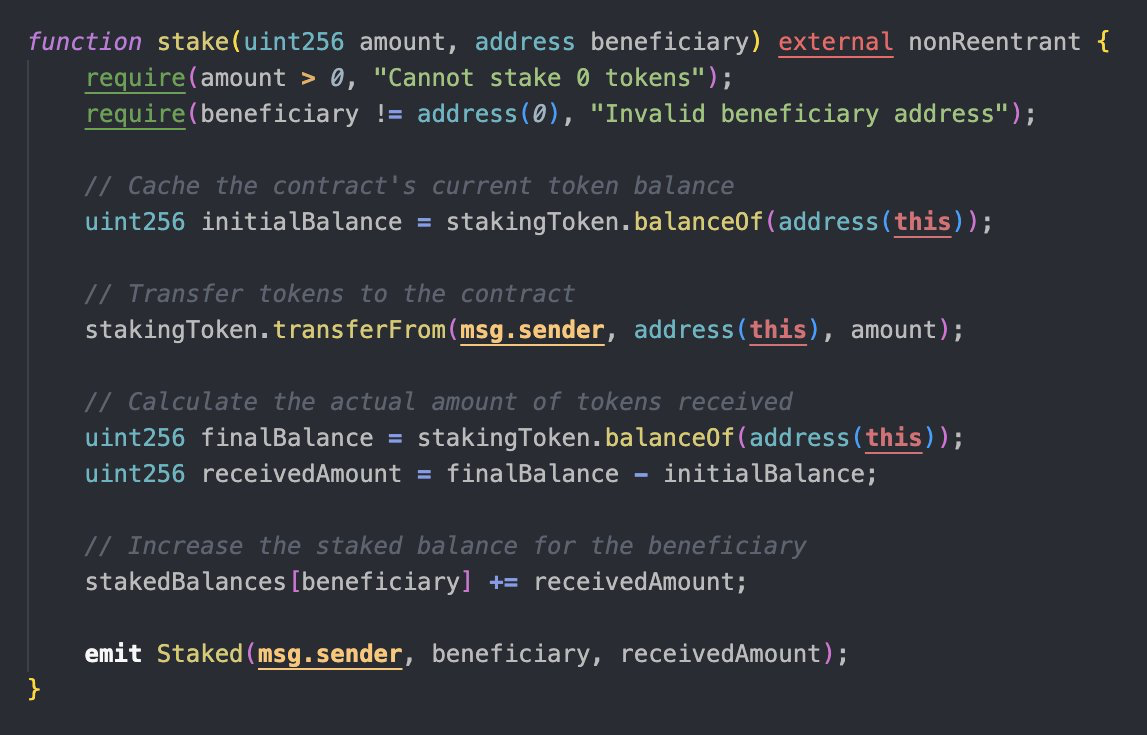