Understanding the Storage Architecture of Solidity Smart Contracts
Introduction
In Ethereum smart contracts, storage is the long-term memory where state variables persist across transactions. Unlike in-memory variables that last only for a function’s execution, storage variables maintain their values throughout the contract’s lifetime. Understanding how Solidity arranges these variables—often referred to as the storage layout—is crucial for optimizing gas usage, ensuring upgradeability, and preventing bugs in advanced scenarios.
This article will explore how Solidity assigns storage slots to different data types, referencing a code snippet with uint256 number, address owner, a Data struct, and a mapping(address => uint256) balances. By the end, you’ll grasp how variables are laid out in memory, why each slot matters, and how you can leverage this knowledge for more secure and efficient contracts. If you need expert guidance or an in-depth security review, consider Bailsec’s audit services.
1. Storage Slots: The Building Blocks
Ethereum contracts store data in storage slots. Each slot is 32 bytes wide (256 bits). Solidity assigns slots sequentially, starting from slot 0 for the first declared variable, slot 1 for the second, and so on. However, complexities arise when dealing with structs, arrays, or mappings—each of which can consume additional slots or compute them dynamically.
Why Slots Matter
- Gas Optimization: Minimizing slot usage can reduce gas costs, as reading and writing to fewer slots is cheaper.
- Upgradeability: In proxy patterns or upgradable contracts, changing a contract’s storage layout can break existing data unless carefully managed.
- Security: Overlapping storage can cause severe vulnerabilities if two variables share the same slot. Thoroughly understanding the layout helps avoid these pitfalls.
2. Example Code Snippet
Below is a simplified version of the code snippet that shows how various variables might be declared:
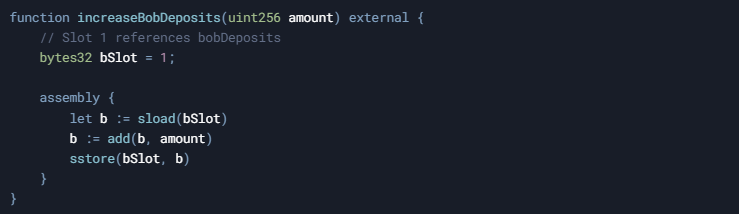
We’ll examine how each of these state variables is placed in storage.
3. Slot-by-Slot Analysis
3.1. Slot 0: number (uint256)
The number variable is a uint256, which occupies a full 32-byte slot. Because number is the first declared variable, it resides at slot 0. A uint256 precisely fits into one slot, so there’s no leftover space in this slot.
3.2. Slot 1: owner (address)
The owner variable is an address, which is only 20 bytes. However, each storage slot is 32 bytes, so the remaining 12 bytes in slot 1 go unused. Solidity doesn’t pack owner with number because they are declared in separate lines, and number already occupies its own slot.
3.3. Slot 2 and 3: data (Struct)
The Data struct has two members, each a uint256 (value and value2). When Solidity stores a struct, it places the first member in the next available slot. For data:
- Slot 2: Holds data.value
- Slot 3: Holds data.value2
Hence, the struct spans two slots because each uint256 requires 32 bytes.
3.4. Slot 4 (Dynamic Allocation for Mapping)
The balances variable is a mapping(address => uint256). Mappings in Solidity do not store their data in a contiguous set of slots like arrays do. Instead, each key-value pair is located at:

Here, slot is the slot number of the mapping’s declaration—in this case, slot 4. For any address addr, the stored value can be found at keccak256(addr, 4). This approach allows for O(1) lookups without wasting space on empty keys.
4. How Solidity Packs Variables
Solidity tries to pack variables within a single slot if they fit. This packing primarily applies to smaller data types (e.g., bool, uint8, or bytesN), which can share a slot until the 32 bytes are filled. However, in the snippet above:
- uint256 fully occupies a slot.
- address also occupies a slot but leaves 12 unused bytes.
- The struct’s uint256 members each require a slot.
If you had declared multiple smaller data types on the same line, Solidity might pack them into one slot, thus saving gas. But in this example, each variable is declared in a way that yields separate slots.
5. Implications for Developers
5.1. Gas Costs
Each slot read or write can cost significant gas. For example, reading a slot costs 2100 gas, and writing to a slot costs 20,000 gas (modulo certain optimizations). Minimizing slot usage or packing variables can reduce costs. However, always weigh clarity against optimization—excessive micro-optimizations can hurt maintainability.
5.2. Upgradeable Contracts
In proxy-based upgradeable contracts, changing the order or type of state variables can corrupt stored data. If you reorder variables or add new ones in the middle, you might shift subsequent variables into the wrong slots. Understanding how your data is laid out—and using carefully planned “storage gaps”—is essential for safe upgrades.
5.3. Security Audits
Auditors often check the storage layout to ensure no overlap or misuse. For instance, if you have multiple inheritance levels, each parent contract might define variables that could inadvertently share slots with derived contracts. Tools like Slither can analyze your code, but a manual review is often required. If you suspect complexity in your layout, consider Bailsec’s reviews to ensure thorough coverage.
6. Best Practices
- Explicit Data Packing
- Group smaller data types (e.g., uint8, bool) together to reduce wasted space.
- Use Structs Wisely
- Keep in mind that each uint256 in a struct typically consumes a full slot. If you have smaller types, they might pack together within the struct’s slots.
- Avoid Unused Bytes
- Although an address can’t share a slot with a uint256 declared on a separate line, you can be mindful of leftover space if you combine smaller types in a single slot.
- Document Storage Layout
- Maintain a clear reference for which slot each variable occupies, especially if you plan on upgrading the contract.
- Don’t Over-Optimize
- Code readability and correctness should trump micro-optimizations. Overly intricate packing can lead to confusion and bugs.
7. Example: Checking a Mapping Entry
To illustrate how mapping keys are stored, consider:

Since balances starts at slot 4, any address key user will have its balance stored at keccak256(abi.encodePacked(user, 4)). This is the location in storage where balances[user] is found. A malicious or advanced user might exploit or manipulate data if your code incorrectly references this slot, so ensure your logic is consistent.
Conclusion
Understanding Solidity’s storage architecture is vital for efficient, secure, and upgradeable smart contracts. Each variable in your contract occupies one or more slots—with simple types like uint256 or address typically filling a single slot, while structs and mappings require additional or dynamically computed slots. By grasping how these slots are assigned, you can:
- Optimize gas usage by carefully packing variables.
- Safely manage upgradeable contracts by avoiding accidental slot overlaps.
- Enhance security by ensuring you don’t inadvertently overwrite critical data.
If your contract has complex data structures, multiple inheritance layers, or a proxy-based upgrade pattern, a thorough understanding of storage slots is indispensable. For expert guidance or a professional security review, visit Bailsec’s services. And for more insights into Solidity best practices, check out our blog.
Disclaimer: This article is for informational purposes only and does not constitute legal or financial advice. Always conduct extensive testing and consider professional audits to ensure your smart contracts meet robust security standards