In Solidity, type casting refers to the process of converting a value from one data type to another, often this is done for gas-saving purposes because smaller uint sizes will then fit into one storage slot. However, when done incorrectly, it can lead to unexpected behavior.
Lets take a look at the following example:
Lets take a look at the following example:
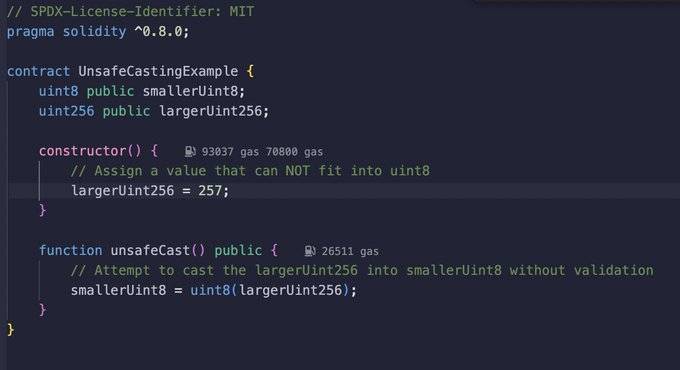
We have a contract named UnsafeCastingExample with two state variables: smallerUint8 of type uint8 and largerUint256 of type uint256. In the constructor, we assign the value 257 to largerUint256. This value is larger than what a uint8 can represent. The unsafeCast function attempts to cast the value of largerUint256 into smallerUint8 using uint8(largerUint256). The clue is, this will not revert but silently overflow.
Lets take a look at the result:
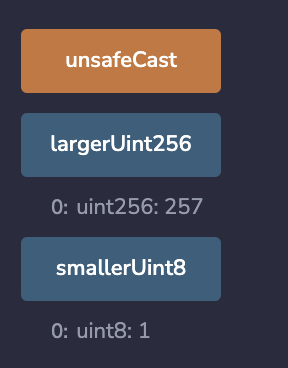
As you can see, the result is 1.
The primary issue with unsafe casting in this example is the risk of overflow. When we cast 257 from a uint256 into a uint8, we exceed the maximum representable value for a uint8, which is 255. This results in an overflow, and the value stored in smallerUint8 will not be 257 as expected, but rather a different value due to the overflow, in that scenario 1.
To avoid such issues, it's crucial to perform safe casting by validating that the value being cast can fit within the target data type's range, this can efficiently done using libraries like SafeCast from OpenZeppelin.