In Solidity, the try/catch statement provides a structured way to handle exceptions and errors that may occur during the execution of external function calls and contract creations. It allows developers to gracefully handle exceptional conditions that may arise during the execution of smart contracts.
try Block: The try block contains the code that may potentially throw an exception or error. This block typically consists of an external function call or a contract creation.
catch Blocks: The catch blocks follow the try block and specify how to handle different types of exceptions or errors that may occur within the try block. Solidity supports multiple catch blocks, each with its own error type or pattern to match.
When using try/catch, only exceptions that occur within the external call itself are caught. Any errors in the expression outside the external call will not be handled by the try/catch mechanism. This means that any computation or state change that happens before the external call will not be covered by the catch block if it fails.
try Block: The try block contains the code that may potentially throw an exception or error. This block typically consists of an external function call or a contract creation.
catch Blocks: The catch blocks follow the try block and specify how to handle different types of exceptions or errors that may occur within the try block. Solidity supports multiple catch blocks, each with its own error type or pattern to match.
When using try/catch, only exceptions that occur within the external call itself are caught. Any errors in the expression outside the external call will not be handled by the try/catch mechanism. This means that any computation or state change that happens before the external call will not be covered by the catch block if it fails.
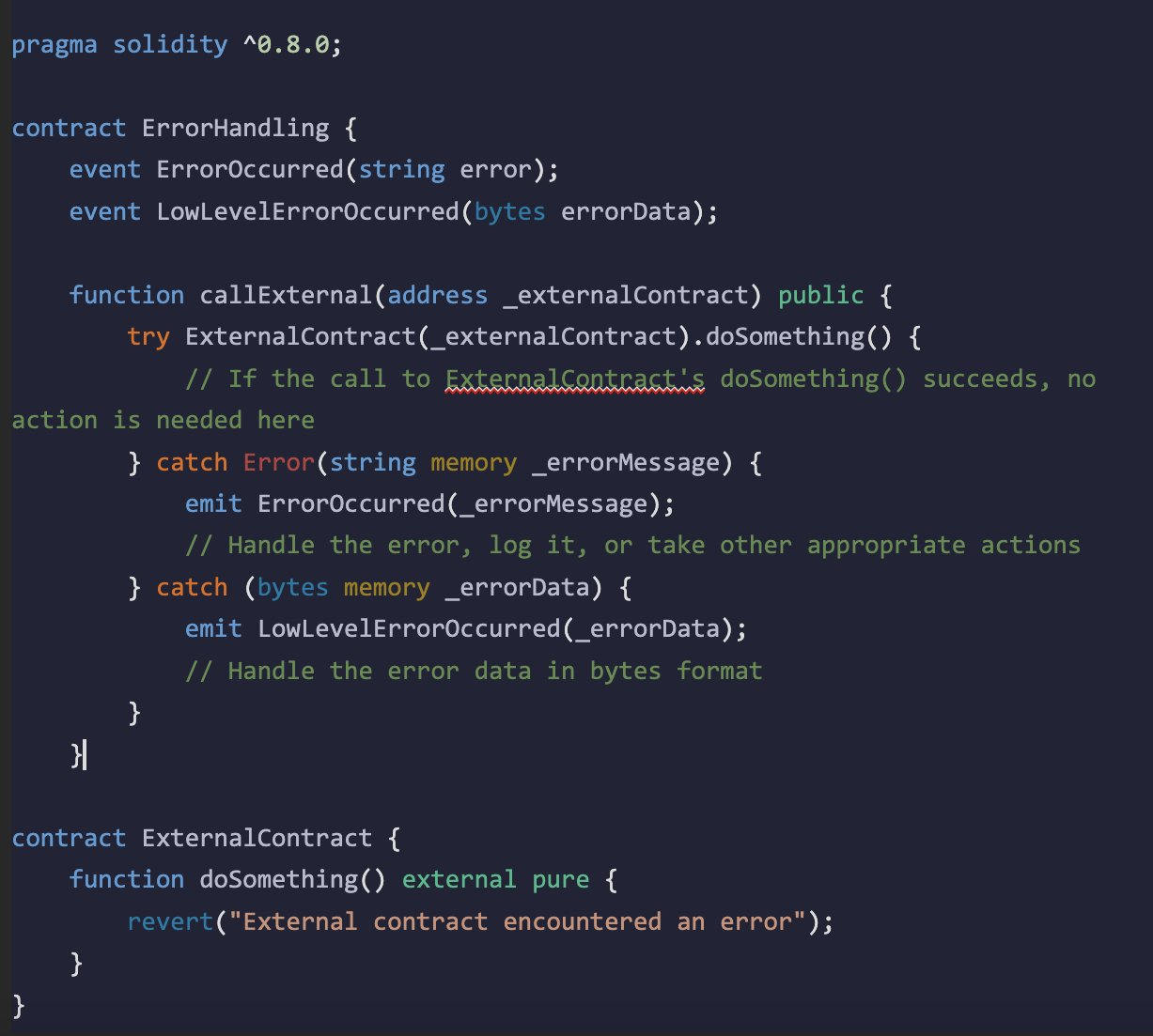
Retrieving the Error Message
In Solidity, the try/catch mechanism allows developers to handle exceptions and errors in 3 major ways.
catch Error(string memory reason):
This catch block is specifically designed to capture and handle errors that are thrown with a revert reason string. In Solidity, functions can revert with an error message using the require, assert, or revert statements. When an external function call fails and provides an error message as a string, the catch Error(string memory reason) block catches this error.

catch Panic(uint256 errorCode)
This catch block captures low-level panics. A panic indicates that the code encountered a condition that should never occur, and it usually means a serious problem. These errors are typically generated by low-level issues such as out-of-gas exceptions or invalid opcode errors or arithmetic reverts.

catch (bytes memory data)
The catch (bytes memory data) block in Solidity is used to capture all errors that do not match the specific patterns of the other catch blocks (catch Error(string memory reason) and catch Panic(uint256 errorCode)). This includes situations where a contract reverts without providing a specific error message.

Critical misuse of Try/catch
One of the most critical misuses is employing empty catch blocks. When a catch block is left empty, errors occurring within the try block are effectively ignored. Interestingly, remix warns about this during compilation.