Introduction:
In the realm of Ethereum smart contracts, efficient data management is key. The low-level calldatacopy instruction in Solidity is designed for precise and optimized copying of transaction call data into memory, reducing overhead and saving gas.
What is calldatacopy?
calldatacopy is a fundamental EVM instruction that copies a specified number of bytes from the transaction’s call data to a designated memory area. It is defined as:
calldatacopy(destOffset, calldataOffset, length)
- destOffset: Memory location to begin copying.
- calldataOffset: Starting point in the call data.
- length: Number of bytes to copy.
Why Use calldatacopy?
Using calldatacopy allows developers to:
- Optimize Gas Usage: Bypass high-level functions for direct memory operations.
- Enhance Performance: Quickly access specific segments of call data.
- Improve Data Handling: Efficiently manage dynamic data in complex contracts
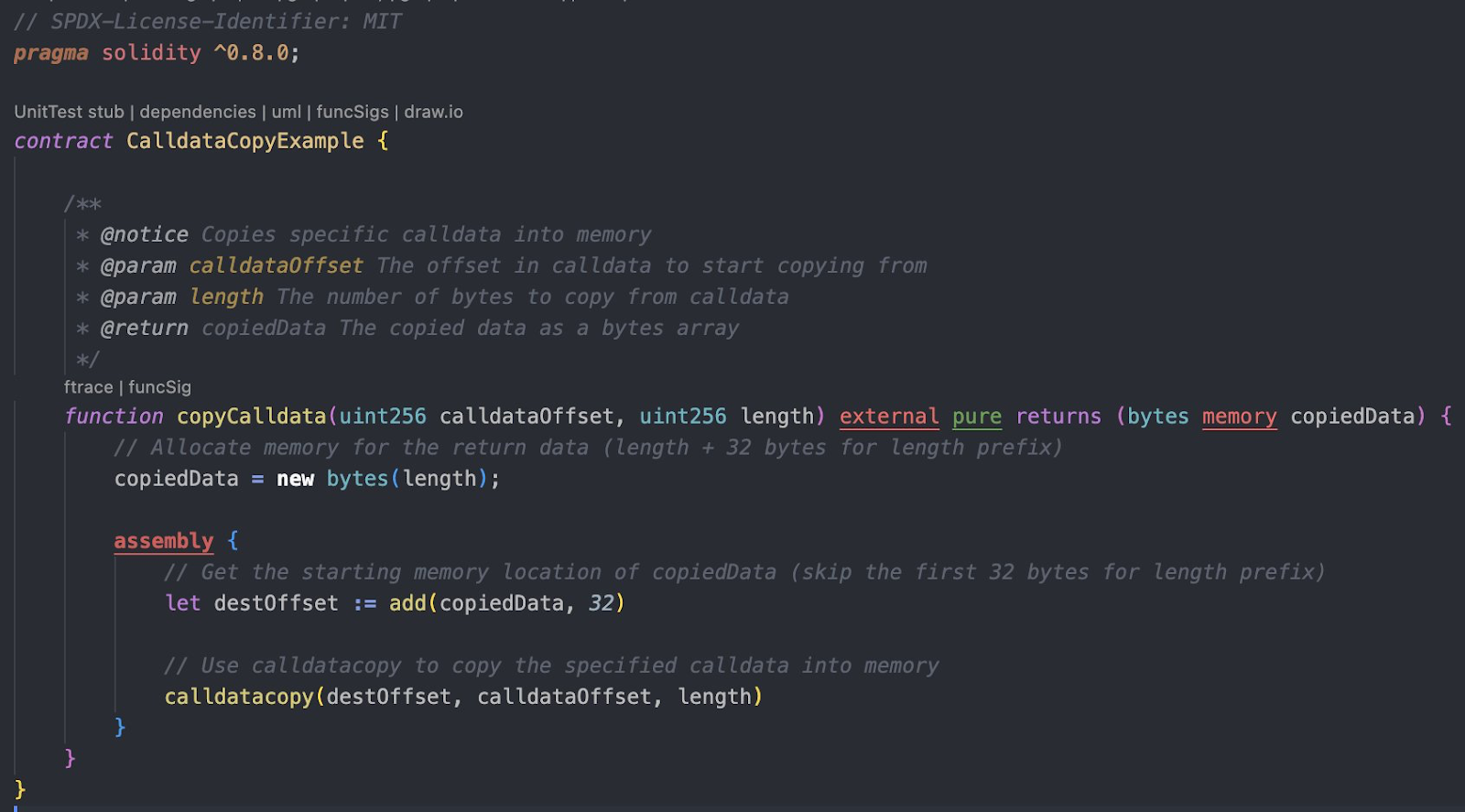
Detailed Example and Explanation
- Memory Allocation:
- When creating a dynamic bytes array, the first 32 bytes in memory store the array’s length. The actual data starts immediately after.
- Setting the Destination Offset:
- In inline assembly, the destination is calculated with add(copiedData, 32), ensuring the array’s length space is skipped.
- Data Copying Process:
- The operation copies the defined length of bytes from the specified calldata offset into memory at the destination offset.
- Return of Copied Data:
- After copying, the new bytes array containing the selected call data is returned for further processing.
Conclusion
Mastering calldatacopy in Solidity is essential for developers aiming to build efficient and cost-effective smart contracts. Implement this instruction to streamline data handling, optimize performance, and reduce gas fees in your Ethereum projects.