In Solidity, assert() is a function used to check for invariants within the code. An invariant is a condition that should always hold true throughout the execution of the contract.
If an assert() statement evaluates to false, indicating that the invariant has been violated, the contract execution is halted, and any remaining gas is not refunded to the caller.
This mechanism is crucial for detecting and preventing unexpected states in the contract, potentially due to bugs or external factors.
This behavior helps prevent the contract from entering an invalid state and ensures that resources are not wasted on operations that cannot proceed.
While assert() is powerful for catching internal errors and ensuring that invariants hold, it should not be used for validating external inputs or conditions that depend on external factors.
For input validation, require() is the appropriate function to use and it is seen as the industry standard.
Here’s an example contract demonstrating proper use:
If an assert() statement evaluates to false, indicating that the invariant has been violated, the contract execution is halted, and any remaining gas is not refunded to the caller.
This mechanism is crucial for detecting and preventing unexpected states in the contract, potentially due to bugs or external factors.
This behavior helps prevent the contract from entering an invalid state and ensures that resources are not wasted on operations that cannot proceed.
While assert() is powerful for catching internal errors and ensuring that invariants hold, it should not be used for validating external inputs or conditions that depend on external factors.
For input validation, require() is the appropriate function to use and it is seen as the industry standard.
Here’s an example contract demonstrating proper use:
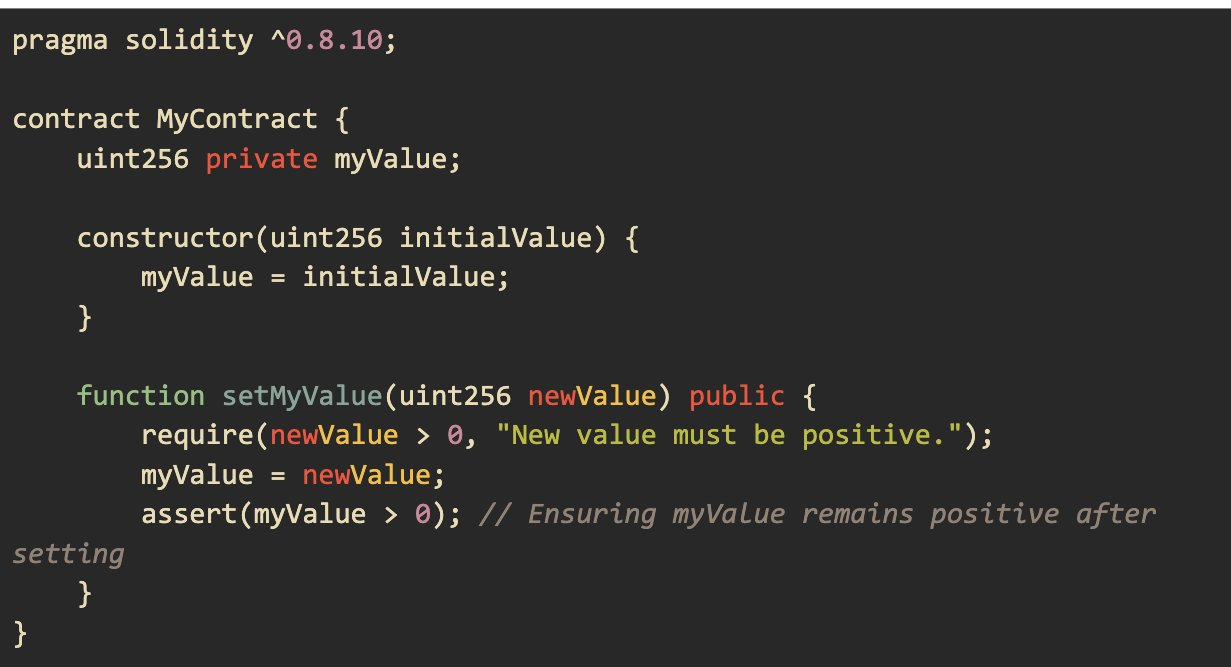
In this example, setMyValue uses require() to validate that the new value is positive before updating myValue. Afterward, assert() is used to ensure that myValue remains positive, acting as a safeguard against unexpected state changes.
The Risks of Misusing assert()
High Gas Costs: When an assert() statement fails, it consumes all the remaining gas in the transaction. This can be extremely costly and inefficient, particularly in scenarios where gas optimization is critical. In contrast, require() returns the remaining gas, making it a more efficient choice for input validation.
Inappropriate Usage: Using assert() for input validation goes against its intended purpose. assert() is meant to catch internal errors, not to validate inputs or external conditions. Input validation should be handled by require(), which is specifically designed for this purpose.
Poor Error Messaging: When an assert() statement fails, it does not provide an error message, making it difficult to diagnose and understand the cause of the failure. On the other hand, require() allows for custom error messages, which can significantly aid in debugging and improving code clarity.
Consider this example of misuse:

In this example, if x is less than or equal to 0, the assert() statement will fail, consuming all remaining gas and providing no error message.
Correct usage:

Here, if x is less than or equal to 0, the require() statement will fail, reverting the transaction, returning the remaining gas, and providing a clear error message.
In summary, using assert() for input validation in Solidity is a risky practice that can lead to high gas costs, poor error messaging, and potential vulnerabilities. By reserving assert() for internal error checks and using require() for input validation, developers can write more secure, efficient, and maintainable smart contracts.