Blog Article: Avoiding Gas Limit DoS Attacks – Smart Strategies for Loop Optimization in Solidity
Introduction: The Challenge of Gas Limit DoS Attacks
In Ethereum smart contract development, one of the most subtle yet dangerous vulnerabilities arises from poorly optimized loops. Unbounded loops that process large arrays or mappings can inadvertently exceed Ethereum’s block gas limit, causing a Denial of Service (DoS) condition. This vulnerability often remains hidden during testing with small datasets, only to emerge in production when data has accumulated substantially. As a result, critical functions may become permanently unusable, locking funds or breaking core functionality.
For those looking for expert help in securing your contracts, our security experts at Bailsec provide tailored services—learn more about our comprehensive security offerings through our services page.
Understanding the Block Gas Limit Constraint
Every Ethereum block has a maximum gas limit (currently around 30 million gas units), which restricts how much computation can occur in a single transaction. This safeguard prevents excessive computational demands but also limits the amount of data a contract function can process at once. As your smart contract’s data structures (like arrays) grow, the gas required for each iteration increases, and eventually, the total gas consumption may exceed the block gas limit. When this happens, the function call fails, causing a permanent DoS condition.
Vulnerable Pattern: The Unbounded Loop
Consider the following example of a vulnerable contract:
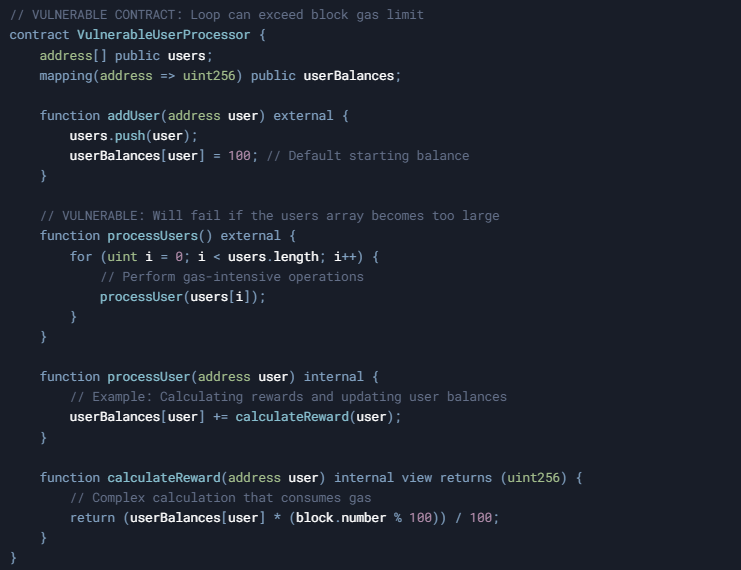
In this contract, as more users are added, the gas cost to execute processUsers() grows linearly. With hundreds or thousands of users, the function may eventually exceed the block gas limit, rendering it uncallable. This not only blocks further functionality but can also lock funds if critical operations depend on processing the entire user base.
For a detailed analysis of vulnerabilities like this, check out our audit services on our security reviews page.
The Secure Solution: Implementing Batch Processing
The most effective solution is to process items in manageable batches rather than all at once. Here’s an improved version of the contract using batch processing:
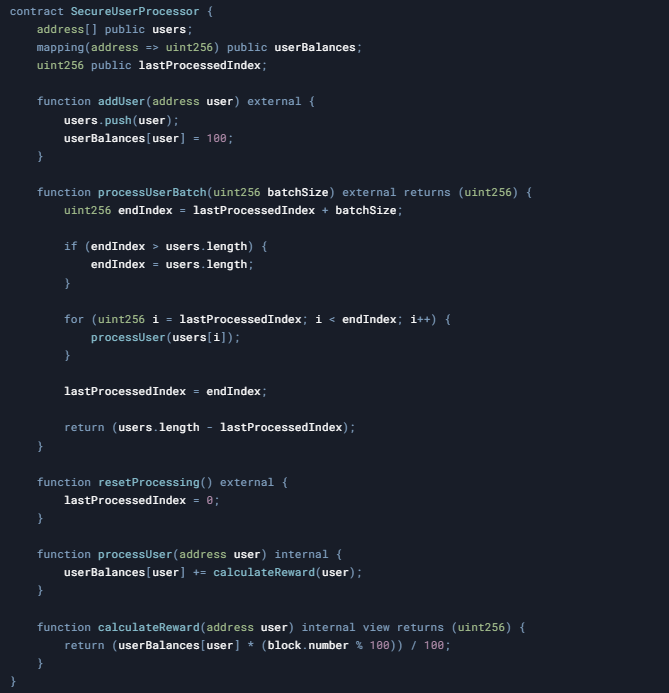
This secure implementation includes several key features:
- Batch Size Parameter: Allows the caller to specify how many items to process in one transaction.
- Progress Tracking: Uses lastProcessedIndex to remember how far processing has progressed.
- Boundary Checking: Ensures that the batch does not exceed the current length of the users array.
- Progress Reporting: Returns the remaining number of items to be processed.
- Reset Capability: Allows restarting the process if necessary.
For more on optimizing contract operations and avoiding DoS risks, review our detailed workflow documentation that explains our secure design strategies.
Advanced Strategies for Loop Optimization
Beyond simple batching, several advanced techniques can further mitigate gas limit issues:
- Pull-Over-Push Payment Pattern:
- Instead of pushing rewards or payments to every user, allow users to pull their rewards individually. This pattern avoids looping over large arrays and reduces the risk of exceeding gas limits.
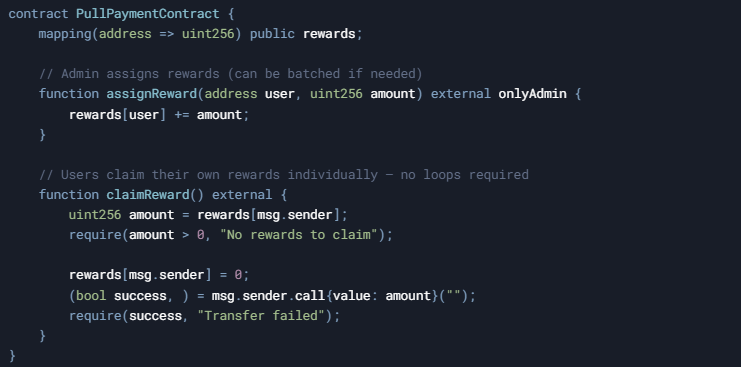
- Pagination with Offsets and Limits:
- Process a specific range of items by specifying a start index and a count, which offers greater flexibility.
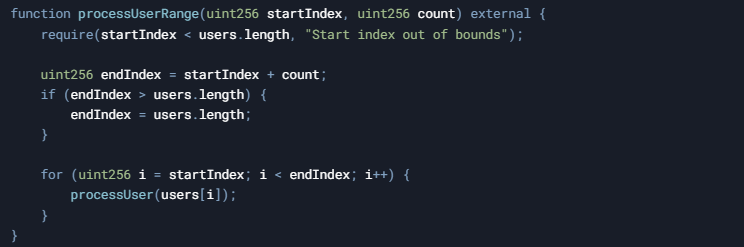
- Optimistic Updates with Checkpoints:
- Update your contract incrementally using checkpoints that record progress and allow the process to resume later.
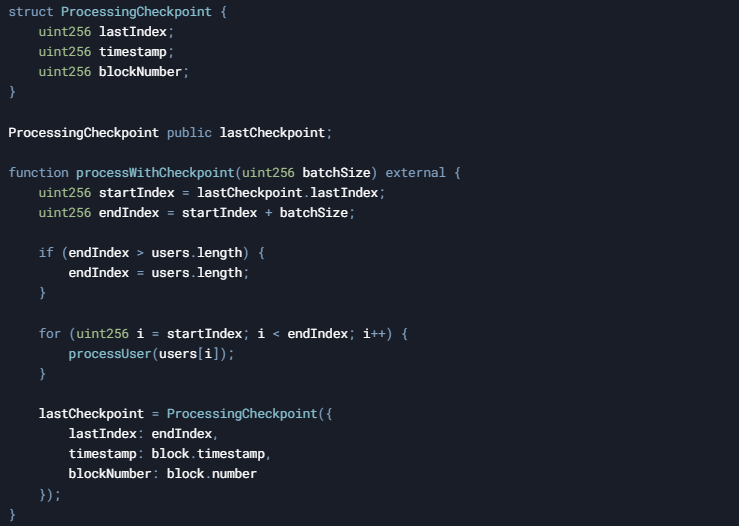
For further examples of secure design patterns, check out our comprehensive guides on our blog where we cover a range of smart contract best practices.
Defensive Gas Estimation
To estimate the optimal batch size, first determine the base gas cost of your function without any loop iterations. Next, calculate the additional gas used for each loop iteration (the per-item gas cost). Then, compute the maximum batch size by subtracting the base gas from the block gas limit and dividing the result by the per-item gas. For example, if your function uses 50,000 gas at base and each iteration uses 30,000 gas, with a block gas limit of 30,000,000 gas, the maximum theoretical batch size would be approximately (30,000,000 - 50,000) divided by 30,000, which is about 999 items. Applying a 20% safety margin to ensure reliability, the safe batch size would be around 799 items.
Our audit services include detailed gas optimization analysis to help determine optimal batch sizes tailored to your contract’s operations.
Monitoring and Circuit Breakers
Even with batch processing, it's prudent to implement monitoring mechanisms and circuit breakers. For example:
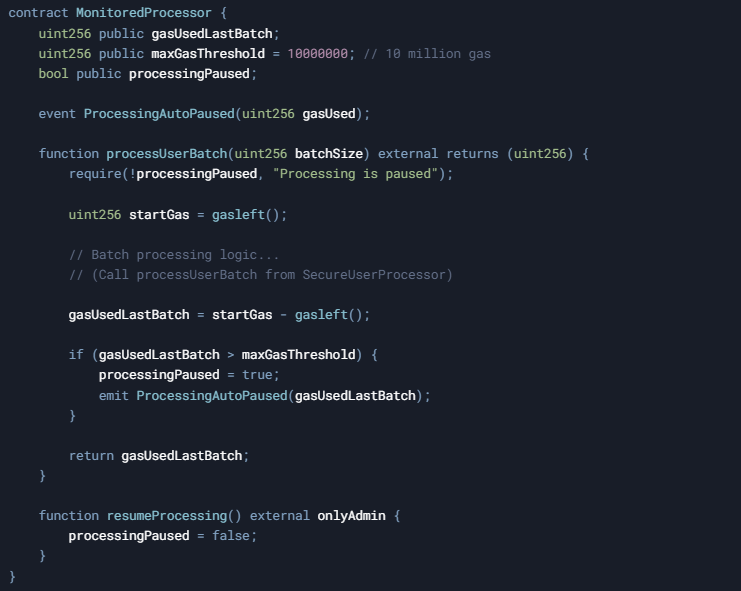
This pattern lets the contract self-monitor gas usage and pause processing if gas consumption exceeds a threshold.
Real-World Examples: Learning from Past Mistakes
Numerous projects have experienced issues related to unbounded loops:
- CryptoKitties Congestion:
- Inefficient loops in breeding and auction mechanisms caused severe network congestion.
- Airdrop Failures:
- Some token airdrops failed because attempting to distribute tokens to too many addresses in one transaction exceeded gas limits.
- DAO Voting Systems:
- On-chain governance systems have sometimes become uncallable as the number of proposals or voters grows too large.
For detailed case studies on these vulnerabilities, read more on our blog where we explore historical incidents and their lessons.
Conclusion: Design for Scale from the Beginning
The key takeaway is to design smart contracts with scalability in mind. Assume that data arrays will grow, user numbers will increase, and attackers may try to push your system beyond its limits. By implementing proper batch processing, using efficient data structures, and carefully monitoring gas consumption, you can build robust contracts that remain functional even as they scale.
In blockchain systems, availability is as critical as security. A contract that becomes unusable due to gas limitations is effectively compromised. For professional assistance in designing gas-efficient and DoS-resistant smart contracts, consider reaching out to the security experts at Bailsec through our services page, where we offer tailored support for your project needs.