A time-locked contract in Solidity is a smart contract mechanism designed to restrict the execution of certain functions until a specified time has passed. This feature is crucial for various applications such as governance mechanisms in DAOs, vesting schedules in DeFi

A timelock is a mechanism in smart contracts that delays the execution of certain functions until a specified period of time has passed. Timelock contracts typically include modifiers or logic that prevent specific functions from being executed until the predetermined time has elapsed.
This ensures that certain actions cannot be taken prematurely, and it is often used in governance systems to delay the decision-making process and its implementation, allowing time for review or potential intervention.
A typical TimeLock contract includes error handling, events for tracking transaction status, constants defining time windows, state variables for storing transaction statuses and owner addresses, modifiers for restricting access, a fallback function for receiving Ether, and external functions for queuing, executing, canceling transactions, and retrieving transaction IDs.
Below is a small snippet of an contract that is deployed a specific amount of ETH and allows the beneficiary to only withdraw the ETH once a specific time has passed:
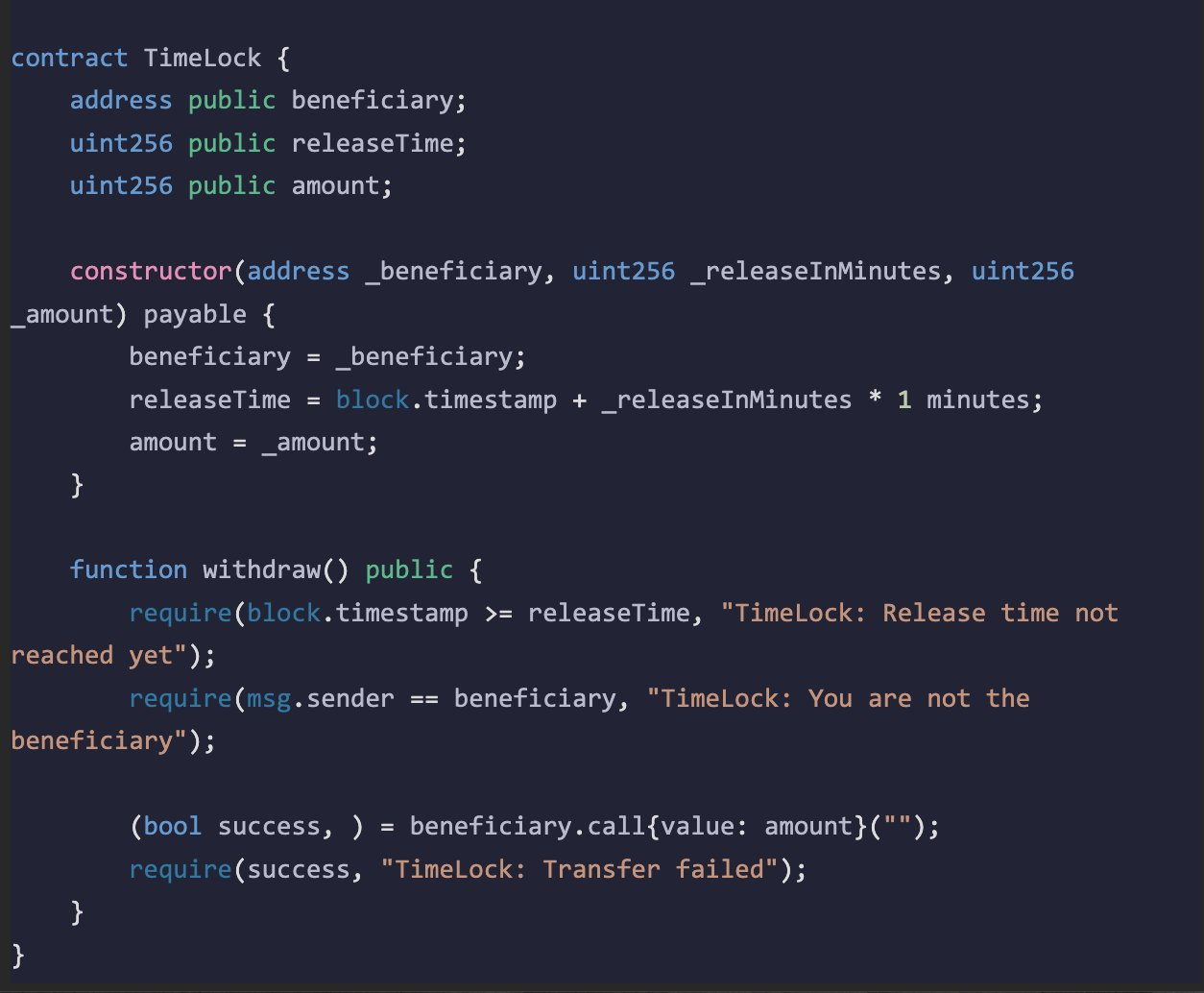