I
Mastering Solidity’s Free Memory Pointer with Bailsec
Introduction: Why the Free Memory Pointer Matters in Solidity
In Solidity, the free memory pointer is a cornerstone of low-level memory management, essential for building efficient and secure Ethereum smart contracts. Located at address 0x40, this pointer dictates where dynamic memory allocation begins in the Ethereum Virtual Machine (EVM). At Bailsec, we recognize its importance for optimizing gas costs and preventing memory-related errors. This guide dives into the mechanics of the free memory pointer, its role in Solidity, and how to manage it effectively using inline assembly.
Understanding the Free Memory Pointer in Solidity
Solidity’s memory is linear and contiguous, with a dedicated section for dynamic storage starting at the address stored in the free memory pointer (0x40). This pointer tells the EVM where the next free memory slot is, enabling efficient allocation for dynamic data like arrays or strings. Unlike persistent storage, memory resets after each transaction, making precise management critical.
The free memory pointer serves two key purposes:
- It ensures efficient memory usage by tracking unallocated space.
- It’s auto-adjusted by Solidity for high-level operations but requires manual handling in inline assembly scenarios.
Missteps in managing this pointer can lead to memory corruption or inflated gas costs – issues our audits at Bailsec are designed to catch and resolve.
How the Free Memory Pointer Works in the EVM
The EVM organizes memory in 32-byte slots, with the free memory pointer at 0x40 initially pointing to 0x80 (after reserved scratch space). When dynamic memory is allocated, the pointer shifts forward – for example, from 0x80 to 0xA0 after storing a 32-byte value. Solidity handles this automatically for standard operations, but low-level tasks like inline assembly demand manual control. At Bailsec, our workflow ensures such operations are executed with precision to maintain contract integrity.
Manually Managing the Free Memory Pointer with Inline Assembly
Inline assembly offers developers fine-grained control over the free memory pointer, crucial for temporary overrides or gas optimization. Consider this practical example:
- Cache the pointer’s value from 0x40.
- Override it temporarily to store three 32-byte values.
- Perform an operation.
- Restore the original pointer value.
Here’s a simplified version in Solidity:
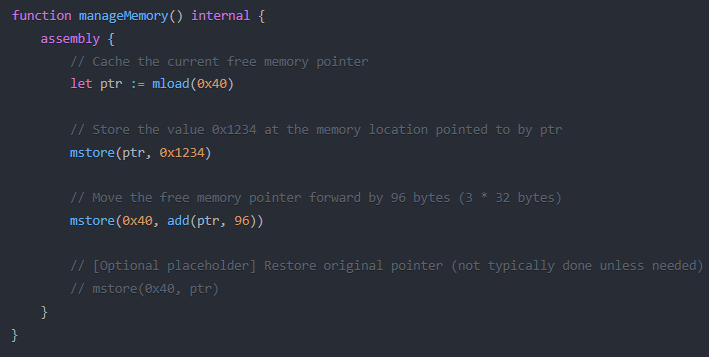
Failing to restore the pointer can corrupt memory, causing subsequent operations to write data at incorrect locations (e.g., arg3 instead of 0x80). Bailsec’s services emphasize restoring the pointer to prevent such risks.
Why Restoring the Pointer Is Critical
If the free memory pointer isn’t reset after manual manipulation, the EVM loses track of free memory. This corruption can crash operations or inflate gas costs as new data overwrites unintended areas. Our audits at Bailsec often uncover such oversights, ensuring your contracts remain robust and efficient.
Benefits for Web3 Developers
Mastering the free memory pointer enhances your ability to write gas-efficient, secure smart contracts – a must for DeFi, NFTs, and other Web3 applications. It reduces costs, prevents errors, and boosts performance. Learn more about optimization techniques in our blog.
Conclusion: Optimize with Bailsec’s Expertise
The free memory pointer is a powerful tool in Solidity when handled correctly. At Bailsec, we help developers master it through expert audits and insights, ensuring your Web3 projects thrive. Ready to optimize your contracts? Explore our services today.
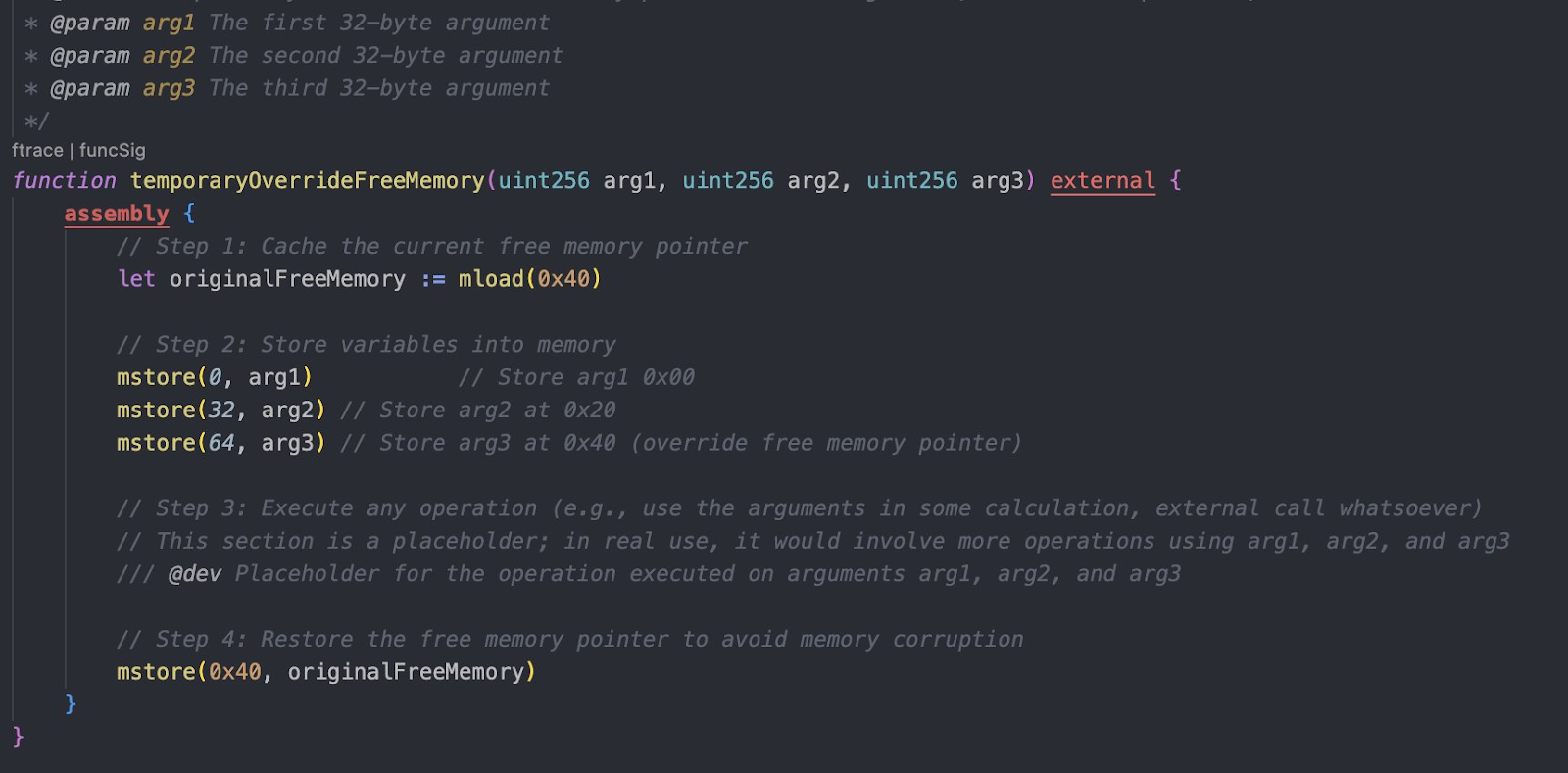