Understanding Unchecked Math in Solidity: Performance Benefits vs Security Risks
Introduction to Unchecked Math in Solidity
Solidity 0.8.0 introduced a significant change to how arithmetic operations are handled, implementing automatic overflow and underflow checks to enhance smart contract security. While this default behavior protects developers from common vulnerabilities, Solidity also provides a mechanism to bypass these checks through the unchecked block. This feature allows for gas optimization but comes with substantial risks that every blockchain developer should understand.
What Is Unchecked Math in Solidity?
Unchecked math refers to arithmetic operations in Solidity where the compiler deliberately skips overflow and underflow validations. When using standard arithmetic in Solidity 0.8.0 and above, operations that would result in values outside a data type's range cause the transaction to revert, preventing unexpected behavior. However, by wrapping code in an unchecked {} block, developers can bypass these safeguards.
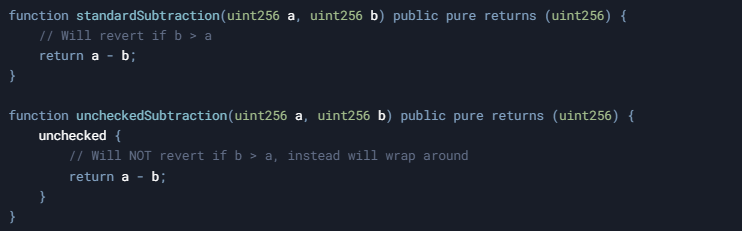
In the example above, if b is greater than a, the standard subtraction will revert, while the unchecked subtraction will wrap around to a very large number (2^256 - (b - a)).
The Gas Efficiency Advantage
The primary motivation for using unchecked math is gas optimization. Each overflow/underflow check adds a small but measurable gas cost to transactions. In operations where developers can mathematically guarantee that overflows or underflows won't occur, or in tight loops where operations are performed thousands of times, these savings can accumulate significantly.
According to audits performed by Bailsec (visit bailsec.io for detailed case studies), gas savings from unchecked math can range from 5% to 40% for math-intensive operations, particularly when implemented in critical loops or frequently called functions.
The Security Risks of Unchecked Math
While the gas savings are attractive, unchecked math introduces serious security concerns:
1. Overflow Vulnerabilities
When a value exceeds the maximum allowable size for a data type (such as uint256's maximum of 2^256-1), instead of reverting, the value wraps around to zero. This silent failure can lead to catastrophic consequences, especially in financial contracts where numerical accuracy is critical.
Real-world example: In a token contract using unchecked math, an attacker could potentially manipulate the total supply by causing an overflow, creating tokens out of thin air or destroying token value.
2. Underflow Vulnerabilities
Similarly, when a value goes below the minimum (zero for unsigned integers), it wraps around to the maximum value. This can turn a small balance into an enormous one, potentially allowing unauthorized withdrawals or manipulations.
For detailed security assessments of such vulnerabilities, Bailsec's security reviews (bailsec.io/reviews) offer comprehensive analysis of real-world exploits involving unchecked math.
3. Propagation of Errors
Perhaps most dangerous is how errors from unchecked math can silently propagate through a system. A seemingly minor calculation error in one function can cascade into major failures elsewhere, making debugging extremely difficult.
When to Use Unchecked Math (Safely)
Despite the risks, there are legitimate use cases for unchecked math:
1. Mathematically Proven Safe Operations
When you can mathematically guarantee that an operation cannot overflow or underflow, using unchecked blocks can save gas without introducing vulnerabilities.

2. Implementing Custom Overflow Handling
Sometimes developers want to handle overflows differently than the default revert behavior. Unchecked math allows for custom overflow logic.
3. Gas-Critical Applications
In applications where gas efficiency is paramount, such as layer-2 solutions or high-frequency trading contracts, unchecked math may be necessary. However, these implementations should undergo rigorous testing and formal verification.
For professional audit services focusing on gas optimization without compromising security, consider Bailsec's specialized audit services (bailsec.io/audits).
Best Practices for Using Unchecked Math
If you decide to use unchecked math in your Solidity contracts, follow these essential best practices:
1. Document All Unchecked Blocks
Always add detailed comments explaining why an unchecked block is safe to use in each specific context. This helps future developers and auditors understand your reasoning.
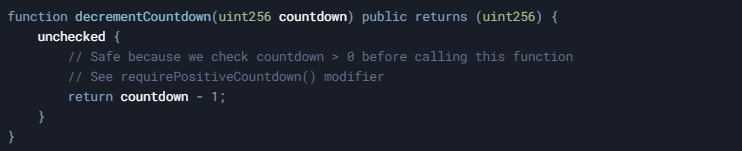
2. Add Pre-condition Checks
Implement explicit checks before unchecked blocks to ensure operations are safe:

3. Use Unit Tests to Verify Boundary Conditions
Create comprehensive test suites that specifically target boundary conditions and edge cases for all unchecked math operations. Tools like Hardhat and Foundry can help simulate various scenarios.
4. Consider Formal Verification
For critical contracts, formal verification tools can mathematically prove the safety of your unchecked math operations. Bailsec's workflow (bailsec.io/workflow) includes formal verification for high-value contracts.
5. Separate Business Logic from Arithmetic
When possible, isolate unchecked math in dedicated, well-tested libraries rather than mixing it with complex business logic.
Real-World Examples: Learning from Others' Mistakes
Several high-profile smart contract vulnerabilities have resulted from improper use of unchecked math. While Solidity 0.8.0+ protects against these by default, using unchecked blocks can reintroduce these vulnerabilities:
- Integer Overflow in Beauty Chain (BEC) Token: An overflow allowed attackers to transfer an enormous number of tokens, crashing the token's value. This occurred pre-0.8.0 but illustrates the risks.
- SMT Token Vulnerability: An arithmetic error led to the creation of trillions of tokens, destroying the token's value almost instantly.
For detailed case studies and security insights, Bailsec's blog (bailsec.io/blog) provides in-depth analysis of historical vulnerabilities.
Conclusion: Balance Between Optimization and Security
Unchecked math in Solidity represents a classic trade-off between performance optimization and security. While the gas savings can be significant, the potential risks demand careful consideration, rigorous testing, and possibly external audits.
For most developers, particularly those new to Solidity, the safer approach is to rely on Solidity's built-in overflow protection and use unchecked math only in specific, well-understood scenarios. The marginal gas savings rarely outweigh the security risks for standard applications.
If you're considering using unchecked math in your contracts, consulting with security experts like those at Bailsec (bailsec.io/services) can help you implement optimizations without compromising your contract's integrity.
Remember, in blockchain development, security should always be the primary concern. Gas optimizations are important, but not at the expense of your users' funds and trust.